- 31 Aug 2024
- 3 Minutes to read
- Print
- DarkLight
SDK: Creating Custom Folder Behavior
- Updated on 31 Aug 2024
- 3 Minutes to read
- Print
- DarkLight
Overview
New Folder behaviors can be created to modify default Folder behavior. Many aspects of a Folder's behavior can be modified. All the overridable methods for a Folder's default behavior are listed at the end of this document. The most common overrides are described below.
Examples
Each example listed will override a member of the DefaultFolderBehavior class.
ShowInTreeView: Overriding lets the user control whether this folder type shows up in the folder tree. By default, it is shown. Here is an example showing how to cause the Folder to be hidden from the tree.
public override bool? ShowInTreeView() => false;
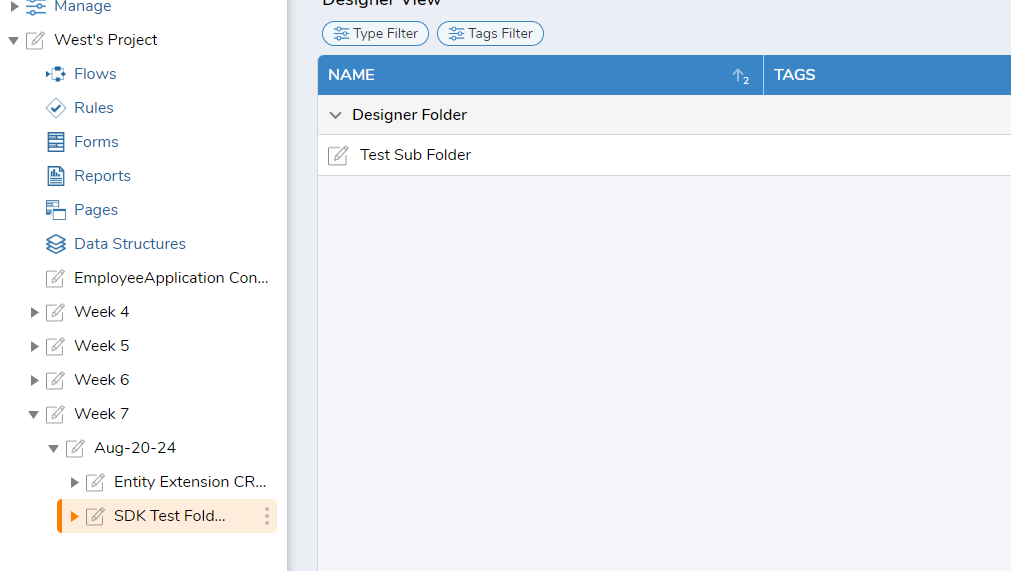
GetFolderActions: Overriding allows the user to control which actions are shown on a Folder. In the example below, in addition to the default action that is supplied for a folder, an action is added to open the URL www.decisions.com.
public override BaseActionType[] GetFolderActions(Folder folder, BaseActionType[] proposedActions, EntityActionType[] types)
{
List<BaseActionType> actions = new List<BaseActionType>((proposedActions ?? new BaseActionType[0]));
actions.Add(new OpenUrlAction("Open Decisions.com", "Clicking this action will open http://www.decisions.com", "http://www.decisions.com"));
return actions.ToArray();
}
.png)
CanBeSetByUser: Overriding allows the user to control whether or not this Folder type is allowed to be added by users via the actions menu. By default, this value is set to false. Below is an example code showing how to override to equal true.
public override bool CanBeSetByUser() => true;
.png)
CanAddEntity: Overriding allows the user to control what entity types are allowed to be added to this Folder type. For example, a user may want to create a Folder type that is only allowed to contain Designer Project Folders. To do this, write an override as follows:
public override bool CanAddEntity(AbstractFolderEntity folderEntity)
{
return folderEntity is Folder && (folderEntity as Folder).FolderBehaviorType == typeof(CustomFolderBehavior).FullName;
}
CanBeRootFolder: Overriding allows the user to control whether or not this Folder is allowed to be a Root Folder. By default, any Folder type other than DefaultFolderBehavior is NOT allowed to be a Root Folder. Setting this override to true will allow the Folder to be a Root Folder. The following sample demonstrates this:
public override bool? CanBeRootFolder() => true;
All Properties that can be Overridden:
public virtual bool AlwaysOpenInNewWindow { get; }
public virtual bool AuditFolderViews { get; }
public virtual bool CanBeDefaultFolder { get; }
public virtual bool CanBeFavorites { get; }
public virtual bool CanBeRecent { get; }
public virtual string FolderBehaviorName { get; }
public virtual string OverrideCreateActionName { get; }
public virtual FolderPermissionBehavior PermissionBehavior { get; }
public virtual bool RecordStateChange { get; }
public virtual bool ShowAlternateViews { get; }
public virtual string SubMenuGroupName { get; }
public virtual void AddToCache(Folder f, AbstractFolderEntity value, bool createIfNotExist = true);
public virtual void AfterBehaviorAssigned(Folder f);
public virtual void AfterDelete(AbstractFolderEntity folderEntity);
public virtual void AfterSave(AbstractFolderEntity folderEntity);
public virtual bool CanAddEntity(AbstractFolderEntity folderEntity);
public virtual bool? CanBeRootFolder();
public virtual bool CanBeSetByUser();
public virtual bool CanBeUnset();
public virtual bool CanChangeNameAndDescription();
public virtual bool CanRemoveEntity(AbstractFolderEntity folderEntity);
public virtual bool CanUserChangeDefaultPage();
public virtual void ClearFolderCache(Folder f);
public virtual void ClearFolderCache(Folder f, Type t);
public virtual void CommitAdditionalFolderData(Folder folder, DataPair[] data);
public virtual bool ContainsStructures();
public virtual DataPair[] GetAdditionalFolderViewData(Folder f);
protected ICache<ConcurrentDictionary<string, AbstractEntity>> GetCache(Folder f, Type t);
protected virtual string GetCacheConfigurationNameForType(Type t);
protected virtual string GetDefaultCacheConfigurationName();
public virtual string GetDefaultPageName(Folder folder);
public virtual BaseActionType[] GetFolderActions(Folder folder, BaseActionType[] proposedActions, EntityActionType[] types);
public virtual string GetFolderEntityDescription(Folder f, string currentDescription, bool forHeader);
public virtual string GetFolderEntityName(Folder f, string currentName);
public virtual Type GetFolderExtensionDataType();
public virtual T[] GetFolderObjects(Folder f, bool includeDeleted = false, bool loadIfNotExists = true) where T : AbstractEntity;
public virtual AbstractFolderEntity[] GetFolderObjects(Folder f, Type t, bool includeDeleted = false, bool loadIfNotExists = true);
public ConcurrentDictionary<string, AbstractEntity> GetFolderObjectsDictionary(Folder f, Type t, bool createAndFetchIfDoesNotExist);
public T[] GetFolderObjectsWithExtensionType<t, extensiontype="">(Folder f, bool includeDeleted = false, bool loadIfNotExists = true) where T : AbstractEntity;
public virtual string GetFolderShortTypeName(Folder f);
public virtual TimeSpan GetLockScreenTimeout(Folder folder);
public virtual bool? GetSendEventsOnAddingToFolder();
public virtual bool? GetSendEventsOnFolderOwnedDataSave();
public virtual bool? GetSendEventsOnFolderSave();
public virtual SubMenuBehavior GetSubMenuBehavior();
public virtual ViewPageData[] GetViewPages(Folder folder);
public virtual bool IsAdditionalFolderViewDataReadOnly(Folder folder, FolderPermission currentUserPermissions);
public virtual void OnBehaviorAssigned(Folder f);
public virtual void OnFolderHidden(Folder f);
public virtual void OnFolderUnhidden(Folder f);
public virtual void OnStateChange(Folder f, string oldState, string newState);
public virtual void OnStateChangeCompleted(Folder f, string newState);
public virtual SubFolderViewData[] OrderSubFolders(SubFolderViewData[] subFolders);
public virtual IORMEntity[] ProcessExportEntities(IORMEntity[] entities);
public virtual void RemoveFromCache(Folder f, AbstractFolderEntity value, bool createIfNotExist = true);
public virtual bool ShouldAutoLockScreen(Folder folder);
public virtual bool? ShowBrowseView();
public virtual bool? ShowInTreeView();
public virtual void UpdateCacheItem(Folder f, AbstractFolderEntity value, bool createIfNotExist = true);